Functions
Studio Functions offers self-service function management that can be used to map a task based on custom, preconfigured JavaScript code that will execute a specific action in your Studio flow.
In this tab, you can configure a Function that enables you to create, test, and publish custom code that will later be used in your Studio call flows, as a regular flow step, through the “Run Function” component.
To configure a Function, follow these steps:
![[Update] Studio - Functions | Limitations..png 2880](https://files.readme.io/f611dbc-Update_Studio_-_Functions__Limitations..png)
1. Once on the Studio Flows page, click on the Functions tab, which will take you to the “Functions Manager” page [1].
2. Click on Create function [2] to open the “New function” form. In this case, we will create a function that will remove the country code from a phone number.
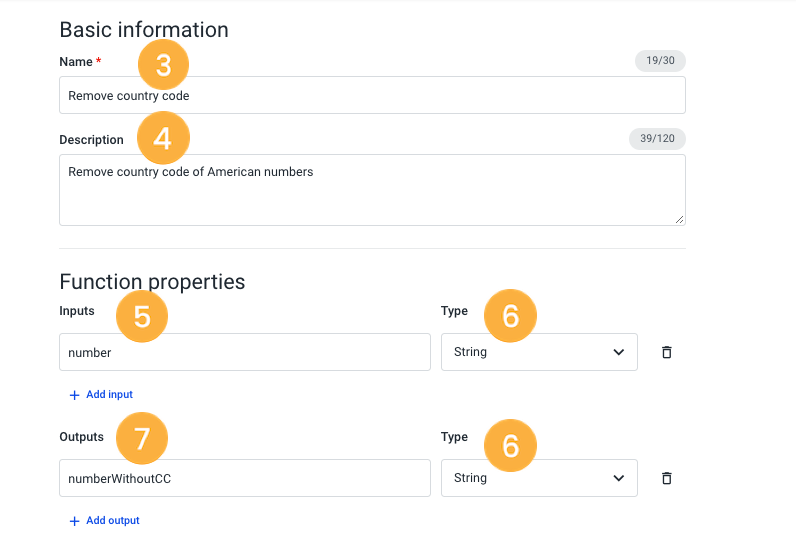
3. Provide a unique Name [3] and a description of the function [4].
4. Enter the input parameter in the “Input” field [5]. This parameter corresponds to the data that is passed to the external data source.
5. Select the type of input parameter from the options on the dropdown - String, Boolean, Integer, Number, URI [6]. These are the only valid input types, which means that complex data types such as objects or arrays are not allowed.
6. Add the desired output, which will return the results that have been requested through the previous field [7].
7. Select the type of output parameter from the options on the dropdown - String, Boolean, Integer, Number, URI [6]. The output parameters present the same type of limitations as the input parameters.
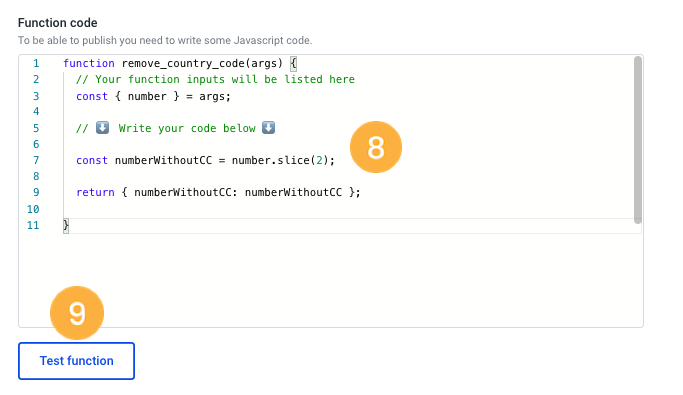
8. Complete the “Function Code” field [8]. This field will receive the function’s name and inputs defined above, following the accepted syntax. These automatically created code lines cannot be changed. The function will also receive specifically one argument, named args. This is an object that contains the inputs defined for the function as keys, and the value will be the variable configured in the flow. You will also need to enter additional JavaScript code in this field, which should be compliant with the example shown below. The value to be returned should be an object, where each key is an output of the function, and the value should be of the defined type.
function(args) {
const { number } = args;
const numberWithoutCC = number.slice(2);
return { numberWithoutCC: numberWithoutCC };
}
9. When finished, you can click Save to create a draft version of your function and then Publish to enable your function. However, we do advise that the function is tested first, to guarantee its proper functioning. To do so, click on Test function [9] button.
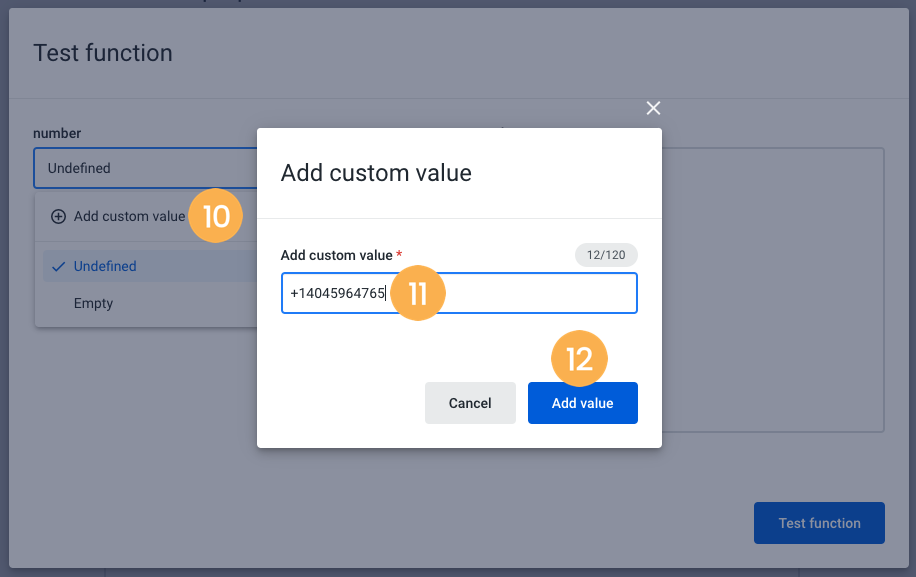
10. When the “Test function” form window is displayed, click on the dropdown and select Custom Value [10].
11. In this case, as we have configured our function to remove the country code, we will enter the phone number with the country code [11], and click on Add value [12].
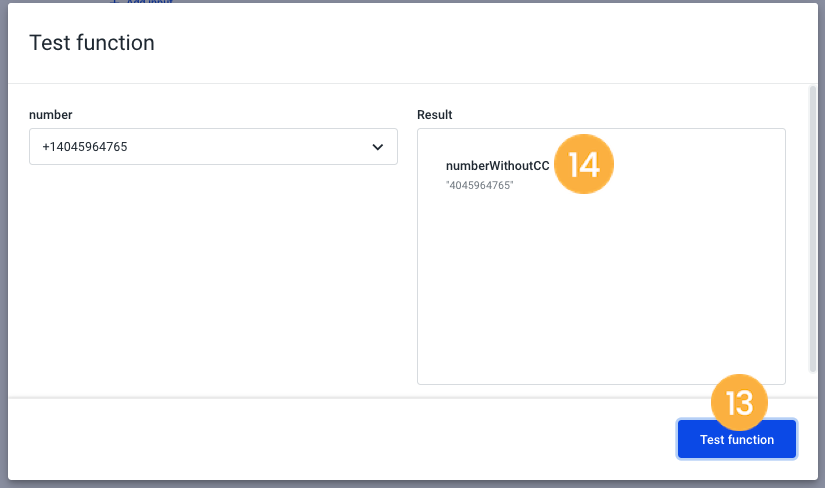
12. Then, click on Test function [13] so the result is displayed [14]. In this case, we can see that the country code “+1” has been removed from the phone number, as expected. We also advise you to test functions over different scenarios to ensure that the expected behavior is retrieved successfully. You can, for example, test the function by using an empty variable when choosing the option "Empty", instead of "Custom value", from the dropdown.
Note
If an exception occurs when running the code, either because JavaScript throws an error (e.g. calling trim on a number variable) or the code explicitly does a throw statement throw new Error();, the Run Function step in the flow will follow the Execution Error exit.
13. If the function does not present any errors, you can enable it by clicking Save and then, Publish.
Editing a Function
Both draft and published functions can be edited. Since published functions can be used in active Studio flows, to mitigate the risk of breaking a flow while editing a function, published functions are limited to adding inputs and outputs; however, the existing inputs and outputs cannot be removed.
Learn how to edit a function by following the steps below:
- Log in to your Talkdesk account and navigate to Studio [1].
- Select Flows [2] and go to the Functions tab.
- Click on the function's card. You can then start editing any field.
- When finished, click Save [3].
- Acknowledge the warning message by ticking the checkbox [4] and confirm by pressing Save [5].
Note
Resources using this function may stop working properly when the function is edited, resulting in critical issues in your contact center operations.
Once the editing process is finished, a backup function is automatically saved with the status “Backup”. The function will have the same name followed by “- copy v1”, where the number will be incremented for each backup created.
Deleting a Function
It is possible to remove a draft or published function.
Note
When deleting a published function, make sure it isn’t being used in any Studio flow.
Deleted functions that are being used in Studio flows will still be executed in those flows.
When editing a Studio flow that uses a deleted function, the Execute Function component must be updated so that a valid function is chosen instead.
To know how to delete a function, please follow the steps below:
- Log in to your Talkdesk account and navigate to Studio [1].
- Select Flows [2] and go to the Functions tab [3].
- Click on the Delete option [4].
- A modal will open for you to confirm your decision. Tick the checkbox to acknowledge the message[5] (this option will only appear if you are deleting a published function).
- Click on the Delete button [6].
Note
Resources using this function may stop working properly when the function is deleted, resulting in critical issues in your contact center operations.
The deleted function will remain visible under the “Deleted” filter. It cannot be changed or used but it can be viewed.
In case of editing a Studio flow where a deleted function is being used, an error message will be presented and a new function must be selected.
Filtering Functions
It is possible to filter functions of specific statuses from the Functions page. By default, only draft and published functions are shown. To show functions in different statuses (deleted or backup), please follow the steps below:
- Click on the status dropdown on the toolbar [1].
- Check the desired function status [2].
- The page will immediately display the functions belonging to the criteria specified through the filters.
Duplicating a Function
Duplicating a function is an easy way to copy a function, instead of having to start from scratch, you only need to change the necessary parts. This option allows speeding the configuration of multiple functions that are similar or creating a newer version of deprecated ones.
To know how to duplicate a function, please follow the steps below:
- Go to the Functions tab [1].
- Click on the Duplicate function option [2]. Then, you can start changing any needed field, as any other Studio Function.
Use Cases
Aside from the example above, Functions can also be used in a variety of ways, as described in the examples below.
Use Case 1: Create a URL that will be sent to an agent through Conversations with the contact's CRM information
Description: Create a URL that will be sent to an agent through Conversations with contact CRM information
Result/Output: A URL with customer information.
http://dummy.com/admin/tickets/new?customerNumber=123&callRef=456
or an error saying:
customerNumber must be a string
Example of the information to fill in on the Functions Manager, following the accepted code syntax:
Function name: URL creation.
Function description: Composes a URL to create a ticket in our CRM with the customer data.
Function input parameters (with type and if it is required or not):
customerNumber: string, required
callRef: string, not required
Function output parameters (with type):
url: URI
Function Code
function(args){
const { callRef, customerNumber } = args;
if(typeof customerNumber !== "string") {
throw new Error("customerNumber must be a string");
}
let searchParams = '?customerNumber=' + customerNumber.trim();
if (typeof callRef === 'string') {
searchParams = searchParams + '&callRef=' + callRef;
}
const url = 'http://dummy.com/admin/tickets/new' + searchParams;
return { url: url };
}
Use Case 2: Alpha-numeric codes for text-to-speech
Description: Returns the input code with each character separated by a space. Useful to override Twillio’s text-to-speech limitations when reading "codes" to the contact (example: “B4GH32” => “B, 4, G, H, 3, 2“).
Result/Output: A new string with the original code separated by spaces at each character: “B, 4, G, H, 3, 2“.
Example of the information to fill in on the Functions Manager, following the accepted code syntax:
Function name: Split with spaces.
Function description: Inserts a space between every character of a string. Useful for reading codes in text-to-speech.
Function input parameters (with type and if it is required or not):
code: string, required
Function output parameters (with type):
spacedCode: string
Function code
function(args) {
const { code } = args;
const codeAsString = String(code);
const spacedCode = codeAsString.split("").join(", ");
return { spacedCode: spacedCode };
}
Use Case 3: Convert a phone number to e164 formatting by adding a ‘+' in front of it
Description: Collecting a customer’s phone number in the IVR with country code first and adding a '+' to it for a Callback.
Result/Output: phone number in e.164 format with the + symbol in front of it.
Example of the information to fill in on the Functions Manager, following the accepted code syntax:
Function name: Add Plus Sign.
Function description: Adds a plus sign to the beginning of a string.
Function input parameters (with type and if it is required or not):
number: string, required
Function output parameters (with type):
numberWithPlus: string
Function Code:
function(args) {
const { number } = args;
const numberWithPlus = '+' + number;
return { numberWithPlus: numberWithPlus };
}
Use Case 4: Concatenate a string of multiple variables to form one full string
Description: combine a ring group name with a language based on a previous menu selection or append a phone number to a URL, etc.
Result/Output: variables will be put in the same string (spacing between characters and/or a symbol like _ is optional and can be customized in the code).
Example of the information to fill in on the Functions Manager, following the accepted code syntax:
Function name: Concatenate Strings.
Function description: Concatenate two strings with a space in-between.
Function input parameters (with type and if it is required or not):
string1: string, required
string2: string, required
Function output parameters (with type):
concatenatedString: string
Function code:
function(args) {
const { string1, string2 } = args;
const concatenatedString = string1 + " " + string2;
return { concatenatedString: concatenatedString };
}
Use Case 5: Remove the country code of US numbers and format the number to (xxx) xxx+xxxx
Description: Collect a customer’s phone number in the IVR with country code, remove it and then format the number to (xxx) xxx+xxxx).
Result/Output: Phone number without the country code and in the correct format, i.e. (385)202+0213.
Example of the information to fill in on the Functions Manager, following the accepted code syntax:
Function name: Remove Country Code and Format Phone Number.
Function description: Removes the country code of American numbers (or numbers with country code of length one) and format the number to (xxx) xxx+xxxx".
Function input parameters (with type and if it is required or not):
number: string, required
Function output parameters (with type):
formattedNumber: string
Function code:
function(args) {
const { number } = args;
const numberWithoutCC = number.slice(2);
const formattedNumber = numberWithoutCC.replace(/^(\d{3})(\d{3})(\d{4})$/, '($1) $2-$3');
return { formattedNumber: formattedNumber };
}
Use Case 6: Play a message to the contact person according to a conditional logic implemented in the Function
Description: Play a message to the contact person informing about the status of the delivery of a specific item and, in some cases, the delivery date as well.
Example of the information to fill in on the Functions Manager, following the accepted code syntax:
Function name: Delivery Status Message
Function description: Construct the message to be played to the contact person informing about the status of the delivery of a specific item and, in some cases, the delivery date as well.
Function input parameters (with type and if it is required or not):
status: string, required
date: string, required
item: string, required
Function output parameters (with type):
messageToPlay: string
Function Code:
cfunction (args) {
const { status, date, item } = args;
const statusInLowerCase = (status || '').toLowerCase();
let str;
switch (statusInLowerCase) {
case 'canceled':
str = `was canceled on ${date}.`;
break;
case 'ready to pickup':
str = `is ready to pick up.`;
break;
case 'shipped':
str = `has been shipped on ${date}.`;
break;
default:
break;
}
let messageToPlay;
if (!str) {
messageToPlay = ''
} else {
messageToPlay = `Your item ${item} ${str}`
}
return { messageToPlay: messageToPlay };
}
Use Case 7: Ability to define a priority for the A&D or Callback components based on a string collected in the IVR
Description: Define a priority based on the ring group/menu selection within a conditional statement to use within an Assignment and Dial component and/or Callback component.
Example of the information to fill in on the Functions Manager, following the accepted code syntax:
Function name: Define priority based on ring group/menu selection.
Function description: Parse priority string and return number instead.
Function input parameters (with type and if it is required or not):
priority: string, required
Function output parameters (with type):
priorityAsNumber: number
Function code:
function (args) {
const { priority } = args;
const priorityAsNumber = parseFloat(priority);
return { priorityAsNumber: priorityAsNumber };
}
Use case 8: Using Data from External APIs Containing Arrays
Please visit this page to learn how to use Functions to configure a Studio Flow that uses the “Execute Action” component to retrieve arrays from an external API.
Limitations
Some JavaScript globals are not available, for example: “setTimeout”, “setInterval”, “require”, or any browser-specific globals such as “console”, “window”, or “document” because the code is not run in a browser.
In general, the following are not currently supported:
- Importing JS/NPM libraries.
- Logging.
- Asynchronous code.
- Execute HTTP calls to external systems (or Talkdesk systems, including other functions).
- Access Talkdesk internal resources and information.
All the necessary context must be provided as function input parameters.
Regarding size, it is recommended to keep the code size under 80kb, which translates to around 80000 characters. However, this number could be different depending on the characters written. For example, the emoji “😀” requires more space than the letter “h”.
Updated over 1 year ago